1.3.C Intro
\(1.3.\)C Intro
1.C compilation
2.Some C grammar
3.C pointer
4.Pointer operation
Why? * Address validity: The pointer is used to store the memory address and point at the specified data. If we cast an integer to a pointer, the integer may not be an valid memory address, and visit this address will lead to program crash. * Type system protection: Cast ignores the protection of the type system. An important function of the type system is to ensure that the operations in your code are safe. For example, converting an integer to a pointer and accessing it, skipping the type system check, might result in accessing an incorrect data type.
5.C string
6.C structures
7.Error types
8.C array
9.Endianness
10.C memory management
For example, the following program is right:
Because the local variable is stored on the stack during the run of
1
2
3
4
5
6
7
8 void functionB(int *ptr) {
printf("Value: %d\n", *ptr);
}
void functionA() {
int x = 10;
functionB(&x);
}functionB
, it's safe to pass its pointer to the function.However, this program is wrong:
After the function is returned, the stack frame where
1
2
3
4
5
6
7
8
9 int* function() {
int x = 10;
return &x;
}
void example() {
int *p = function();
printf("Value: %d\n", *p);
}x
is located is released, and the memory address pointed to by pointerp
may be overwritten by other data. This can lead to undefined behavior
Static string constants are typically stored in read-only memory, and modifying these is an undifined behavior in C.
The main reason for placing 4 bytes of data on multiples of 4 is to optimize the processor's memory access efficiency. Suppose a processor has a 4-byte memory bus width. When accessing 4-byte aligned data, the processor can retrieve the entire data in one memory read operation. If the data is not aligned (for example, an int spanning two memory addresses) , the processor may need to perform two memory reads and then merge the two reads. This can cause performance degradation.
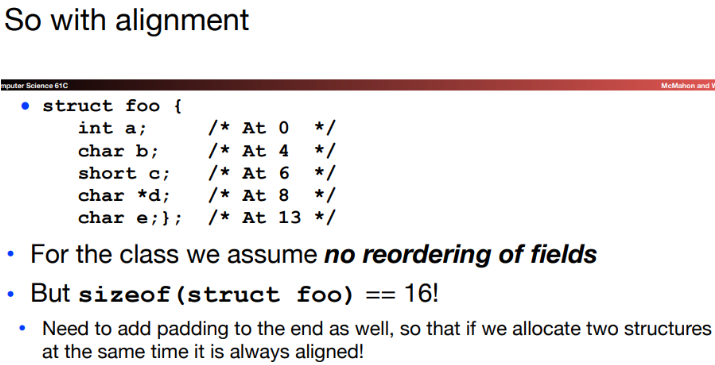
Let's take an example to illustrate the alignment process:
The
1
2
3
4 struct Example {
char a;
int b;
};a
requires 1 byte, and alignment requires 1 byte, so it can be placed at the starting address of the struction.The
b
requires 4 bytes, and alignement requires 4 bytes, so it must be placed on a multiple of 4 address. To satisfyb
's alignment requirements, the compiler inserts three padding bytes aftera
, makingb
's address a multiple of 4.